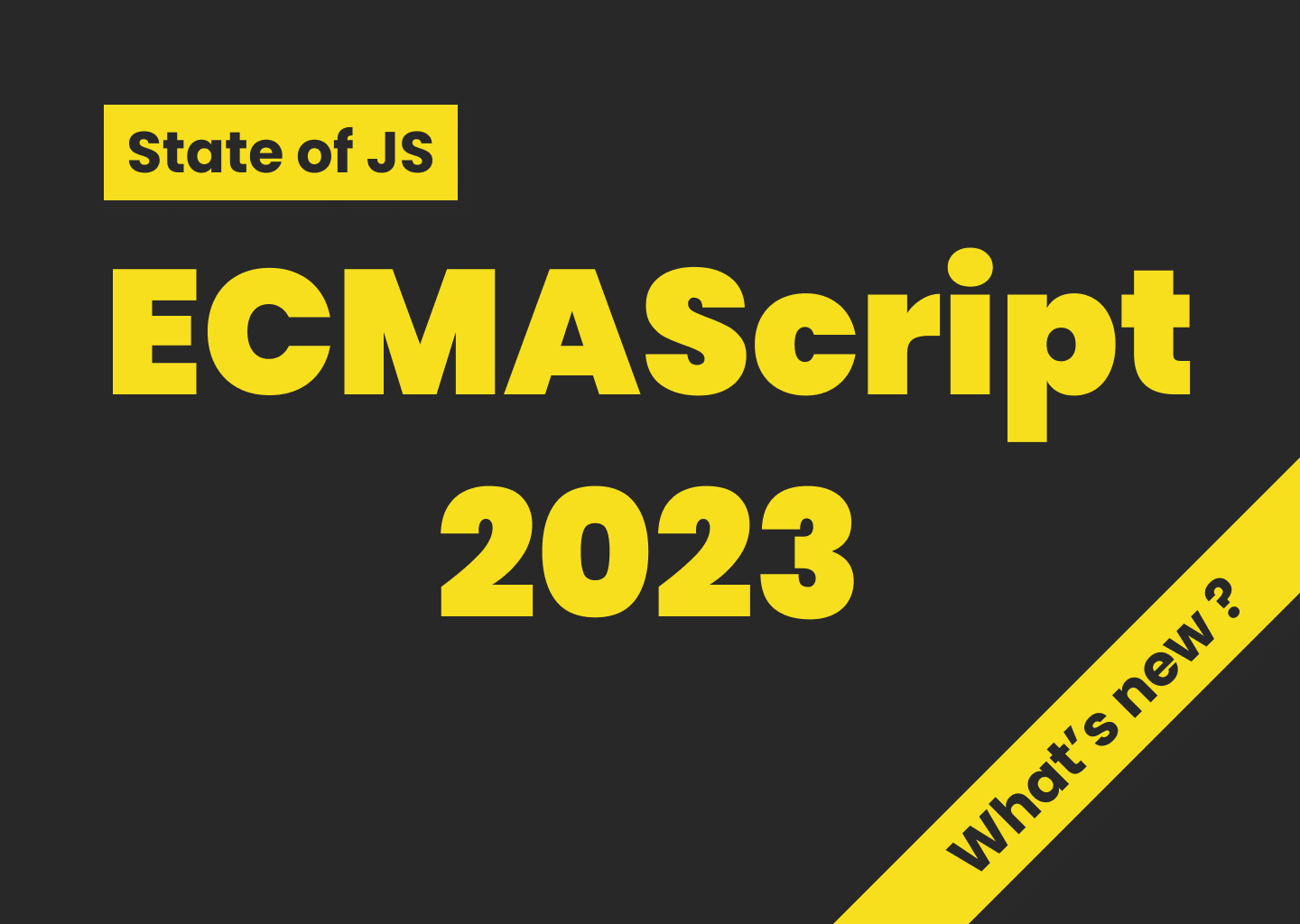
2024-03-31
3 min read
2024-03-31
3 min read
JavaScript has once again evolved with the introduction of ES2023, also known as ECMAScript 2023 or ES14. This latest specification brings a range of new features and methods designed to improve performance and enhance the developer experience. In this blog post, we’ll delve into the key additions in ES2023 and provide explanations, structures, and examples for each one.
These new methods allow developers to search an array from the end, finding the last element that satisfies a given condition. Both functions accept an optional thisArg parameter, which can be used to set the this value for the callback function.
1array.findLast(callback(element, index, arr), thisArg);2array.findLastIndex(callback(element, index, arr), thisArg);
1const fruits = ["apple", "banana", "pear", "orange"];2const lastFruit = fruits.findLast((fruit) => fruit.length === 5); // Returns "orange"3const lastIndex = fruits.findLastIndex((fruit) => fruit.length === 5); // Returns 3
ES2023 formalizes the usage of hashbang (#!) at the beginning of JavaScript files, allowing them to be executed as standalone scripts. This is especially useful for command-line utilities and server-side scripting.
1#!/usr/bin/env node
The Hashbang Grammar formalizes the usage of Hashbangs (also known as Shebangs) at the beginning of JavaScript files.
Hashbangs are directives typically found at the beginning of Unix-based scripts that determine the interpreter for the script. This update allows JavaScript to standardly interpret scripts beginning with a hashbang, specifically in a Node.js environment.
The Hashbang Grammar essentially allows the execution of JavaScript scripts as standalone executables, without the need for an explicit node command. For example:
This JavaScript file can now be executed directly from a Unix-like command line, outputting “Hello, World!” to the console.
The Hashbang Grammar is only valid at the start of a file, and not in other places within the source text. This is to match the de-facto usage in some command-line JavaScript hosts that allow for Shebangs/Hashbangs.
The Hashbang Grammar is supported in various JavaScript engines, including Chrome 74, Firefox 67, ChakraCore, Safari 13.1, and Node.js 12.0.0.
This addition enables Symbols to be used as keys in WeakMaps, providing a safer way to store private data without the risk of memory leaks. Symbols are guaranteed to be unique, ensuring that they won’t conflict with other keys in the WeakMap.
1const map = new WeakMap();2const sym1 = Symbol("key1");3const sym2 = Symbol("key2");4const obj1 = {};5const obj2 = {};6
7map.set(sym1, obj1);8map.set(sym2, obj2);9
10console.log(map.get(sym1)); // Output: {}, obj111console.log(map.get(sym2)); // Output: {}, obj2
Several new methods have been added to Array.prototype and TypedArray.prototype, providing immutable alternatives to existing array methods:
ES2023 brings an exciting range of new features and methods to JavaScript, making the language even more powerful and flexible. By adopting these new additions, developers can write cleaner, more efficient, and more performant code. As JavaScript continues to evolve, staying up-to-date with the latest changes ensures you remain at the forefront of web development.