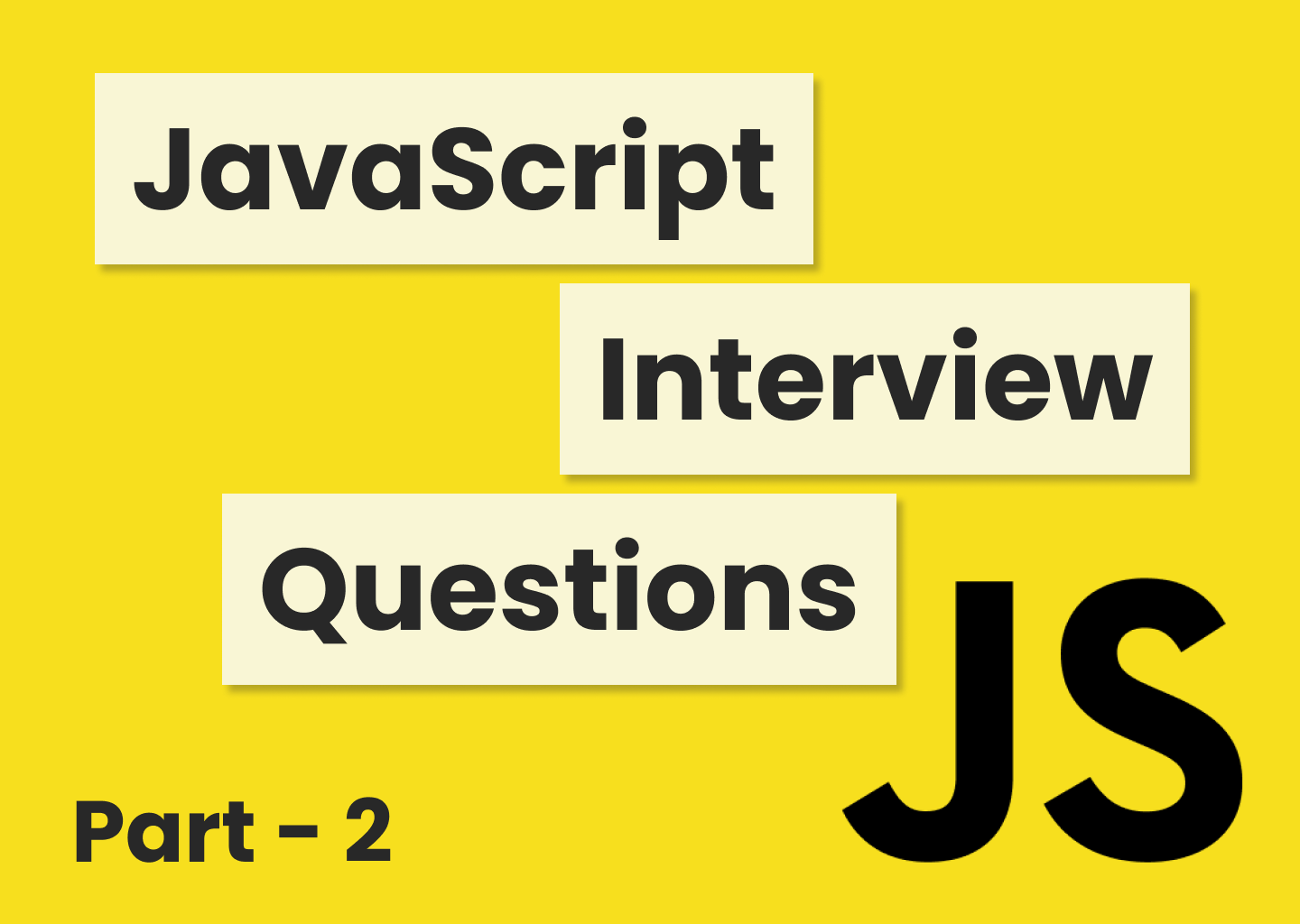
2024-03-29
18 min read
2024-03-29
18 min read
In this post I will list some common JavaScript coding interview questions. This is the sieries of posts, in which I’will list most commont questions with answers and explanations to them. I offer you to try to answer the question by yourself and then look up the answer
1// Example2
3console.log(range(1, 50)); // [1,2,3,4, ... , 50]
1// Answer2const range = (start, end) => {3 const arr = [];4 for (let i = start; i <= end; i++) {5 arr.push(i);6 }7 return arr;8};
This JavaScript code defines a function called range that takes two numbers as input: start and end. The function creates an array and fills it with numbers starting from start and ending with end. Here’s a step-by-step explanation of how it works:
1// Answer2const range = (start, end) => {3 return [...Array(end - start).keys()].map((el) => el + start);4};
This JavaScript code is an alternative implementation of the range function using a different approach. It creates and returns an array containing integers between start and end inclusively.
MDN Array Constructor
MDN .keys()
MDN .map()
What will be logged to the console in first and second examples?
1// Example2const testArr = [1, 2, 3, 4, 5];3
4console.log(shuffle(testArr)); // [2,5,3,1,4]
1// Answer2// A: Fisher-Yates sorting algorithm3const shuffleArr = (arr) => {4 for (let i = arr.length - 1; i > 0; i--) {5 const j = Math.floor(Math.random() * (i + 1));6 [arr[i], arr[j]] = [arr[j], arr[i]];7 }8 return arr;9};
This JavaScript code defines a function named shuffleArr that takes an array (arr) as an input parameter and shuffles its elements randomly. The shuffling is done in-place, meaning the original array is modified. Here’s a detailed explanation of how the code works:
1for (let i = arr.length - 1; i > 0; i--)
1const j = Math.floor(Math.random() * (i + 1));
1[arr[i], arr[j]] = [arr[j], arr[i]];
In summary, the shuffleArr function randomizes the order of elements in the input array (arr) by iteratively swapping each element with a randomly selected element in the unshuffled portion of the array.
1// Answer2const shuffleItems = (items) => {3 return items4 .map((item) => ({ sort: Math.random(), value: item }))5 .sort((item1, item2) => item1.sort - item2.sort)6 .map((a) => a.value);7};
1return items.map((item) => ({ sort: Math.random(), value: item }));
1return items2 .map((item) => ({ sort: Math.random(), value: item }))3 .sort((item1, item2) => item1.sort - item2.sort);
1return items2 .map((item) => ({ sort: Math.random(), value: item }))3 .sort((item1, item2) => item1.sort - item2.sort)4 .map((a) => a.value);
By assigning a random number to each item and sorting the array based on those numbers, the function ensures that each possible permutation of the input array is equally likely.
1//Example2console.log(findMinOccur([1, 1, 2, 1, 3, 5])); // 3
1// Answer2const findMinOccur = (arr) => {3 const minVal = Math.min(...arr);4 return (occNum = arr.filter((item) => item === minVal).length);5};
This JavaScript code defines a function named findMinOccur that takes an array (arr) as input and returns the count of occurrences of the smallest element within the array. The code utilizes built-in JavaScript methods to achieve this functionality. Here’s a breakdown of how the code works:
1const minVal = Math.min(...arr);
1return (occNum = arr.filter((item) => item === minVal).length);
In summary, the findMinOccur function identifies the smallest element within the input array (arr) and returns the count of its occurrences. The function achieves this by finding the minimum value (minVal), filtering the array to find matching elements, and calculating the count based on the length of the filtered array.
1// Example 12function getItem() {3 console.log(this);4}5getItem();
1// Answer2window or undefined in stict mode
When the given JavaScript code is executed, the value logged to the console will be window in normal mode and undefined in strict mode. Here’s the explanation:
In normal mode, the getItem() function is called without any specific context (this) provided. In such cases, JavaScript sets the this value to the global object, which is the window object in a browser environment. So when console.log(this) is executed inside the getItem() function, it logs the window object to the console.
In strict mode, the behavior of this changes when a function is called without a specific context. In this case, this remains undefined instead of referring to the global object.
So when the getItem() function is called in strict mode, console.log(this) logs undefined to the console, indicating that no specific context was provided.
1// Example 22const item = {3 title: "Ball",4 getItem() {5 console.log("this", this);6 },7};
1// Answer2[object];
When the given JavaScript code is executed, the value logged to the console will be Object. This happens because of the way the getItem() method is defined and called within the item object. Here’s the explanation:
1// Example 12class Item {3 title = "Ball";4 getItem() {5 console.log(this);6 }7}8const item2 = new Item();9item2.getItem();
1// Answer2new insance of Item obj
When the given JavaScript code is executed, the value logged to the console will be an instance of the Item class, specifically the object stored in the item2 variable. This happens due to the way the getItem() method is defined within the Item class and how it is invoked on an instance of the class. Here’s the explanation:
MDN this keyword
JsInfo - this keyword
Task 1: Design a class for employee which takes id and name during construction of object and has a salary property.
1// Answer2class Employee {3 constructor(id, name) {4 if (!id || !name) {5 throw new Error("Employee name and id are mandatory");6 }7 this.name = name;8 this.id = id;9 }10
11 getName() {12 return this.name;13 }14
15 getId() {16 return this.id;17 }18
19 setSalary(salary) {20 this.salary = salary;21 }22
23 getSalary() {24 return this.salary;25 }26}27const emlp1 = new Employee(29, "Ole");28
29console.log(emlp1.getName());30console.log(emlp1.getId());31emlp1.setSalary(65000);32console.log(emlp1.getSalary());
Task 2: Create a class of Manager which is employee but can have a department.
1class Manager extends Employee {2 setDepartment(department) {3 this.department = department;4 }5
6 getDepartment() {7 return this.department;8 }9}
1// Answer2const debounce = (fn, delay = 300) => {3 let timer;4 return function (...args) {5 clearTimeout(timer);6
7 timer = setTimeout(() => {8 fn(...args);9 }, delay);10 };11};12
13const inputRes = (arg) => {14 console.log(`The arg is ${arg}`);15};16
17const tryIt = debounce(inputRes, 2000);18
19tryIt("LOL");20tryIt("LOL");21tryIt("LOL");22tryIt("LOL"); // only this one will be executed after 2sec of delay
This JavaScript code demonstrates the concept of debouncing, which is a technique used to delay the execution of a function until a certain amount of time has passed since the function was last called. This is often used in scenarios like handling user input events, such as keypresses, to prevent costly operations from being performed too frequently.
1const debounce = (fn, delay = 300) => {};
1let timer;
1return function (...args) {};
1clearTimeout(timer);
1timer = setTimeout(() => {2 fn(...args);3}, delay);
When calling tryIt multiple times within a short time span, only the last call will be executed after a 2000-millisecond delay, effectively demonstrating the debounce functionality.
1const throttle = (fn, delay = 300) => {2 let isWaiting = false;3 return (...args) => {4 if (!isWaiting) {5 fn(...args);6 isWaiting = true;7 setTimeout(() => {8 isWaiting = false;9 }, delay);10 }11 };12};13
14const inputRes = (arg) => {15 console.log(`The arg is ${arg}`);16};17
18const tryIt = throttle(inputRes, 2000); // Initial call19
20setTimeout(() => {21 tryIt("LOL");22}, 1000); // ignored23
24setTimeout(() => {25 tryIt("LOL");26}, 1500); // ignored27
28setTimeout(() => {29 tryIt("LOL");30}, 2400); // Called
This JavaScript code demonstrates the concept of throttling, which is a technique used to control the execution rate of a function, ensuring that it is called no more than once within a specific time interval. This is often used to limit the rate at which expensive operations are performed, such as API calls or event handlers.
1const throttle = (fn, delay = 300) => {};
1let isWaiting = false;
1return (...args) => {};
1if (!isWaiting) {2 fn(...args);3}
1isWaiting = true;2setTimeout(() => {3 isWaiting = false;4}, delay);
Throttling Demonstration: The code uses setTimeout to schedule calls to the throttled tryIt function with different arguments at different times. The first call happens immediately (tryIt(“LOL”);). The second and third calls are scheduled to happen after 1000 and 1500 milliseconds, respectively. Since these calls occur within the 2000-millisecond throttle delay, they are ignored. The fourth call, scheduled after 2400 milliseconds, is executed, demonstrating the throttling functionality.
1setTimeout(() => {2 tryIt("LOL");3}, 1000); // ignored4setTimeout(() => {5 tryIt("LOL");6}, 1500); // ignored7setTimeout(() => {8 tryIt("LOL");9}, 2400); // Called
In summary, the throttle function ensures that the input function fn is called at most once within the specified time interval delay, thereby controlling the rate of function execution.
CSS-Tricks: Debouncing and Throttling Explained Through Examples
David Walsh Blog: JavaScript Debounce Example
Medium: Understanding JavaScript Debounce
MDN Web Docs: Throttling
Educative: Understanding Debounce and Throttle Functions in JavaScript
Coding Ninjas: Understanding JavaScript Throttling by Building Your Own Throttle Function
Task 1: Highlight all the words longer than 8 characters in the document.
1const textP = document.querySelector("p");2
3textP.innerHTML = textP.innerHTML4 .split(" ")5 .map((word) =>6 word.length > 87 ? `<span style="background-color: yellow">${word}</span>`8 : word9 )10 .join(" ");
Task 2: Add a link to the source after the paragraph element.
1const link = document.createElement("a");2link.href =3 "https://designtechworld.medium.com/everything-about-currying-in-javascript-a2614b82e6ca#:~:text=In%20conclusion%2C%20currying%20is%20a,reusability%2C%20composability%2C%20and%20flexibility.";4link.innerText = "Text for link";5
6document.body.appendChild(link);
Task 3: Split each new sentence to a separate line in the paragraph text. A sentence can be assumed to be a string of text terminated with a period (.)
1textP.innerHTML =2 textP.innerHTML.split(/\.|\!|\?[^.|<]/).join(".</p><p>") + "</p>";
MDN intro to the DOM
1const toDoList = document2 .querySelector(".todo-list")3 .addEventListener("click", (e) => console.log(e.target.innerText));
Event delegation is a technique in JavaScript where an event listener is attached to a parent element rather than individual child elements. When an event occurs on a child element, it bubbles up through the DOM tree, and the parent element’s event listener handles the event. This approach improves performance by reducing the number of event listeners needed. In the provided code snippet, event delegation is used:
This code demonstrates event delegation because a single event listener is attached to the parent .todo-list element, and any click events on its child elements will bubble up to be handled by this single listener. This helps keep the code more efficient and maintainable compared to attaching individual event listeners to each child element.
JsInfo Event delegation
1// Answer2const xhr = new XMLHttpRequest();3xhr.open("GET", "https://asafssaf.com");4xhr.send();5xhr.onload = function () {6 if (xhr.status !== 200) {7 console.log("Error" + xhr.status + xhr.tatusText);8 } else {9 console.log("Succes", xhr.response);10 }11};12
13xhr.onerror = function () {14 console.log("request failed");15};
XML HTTP requests are not used anymore (nowadays we use fetch() or 3rd party solutions like Axios), but this question may appear if you are being interviewed for postition which will include work wiht legacy codebases, so It’s good to know how this worked back in the days.
Here are 10 of most common JavaScript interview coding questions you can face in 2024. This is a series of interview preperation posts. So stay tuned! You can follow me on social media, where I’ll introduce new blog posts as soon as they are published on the website.