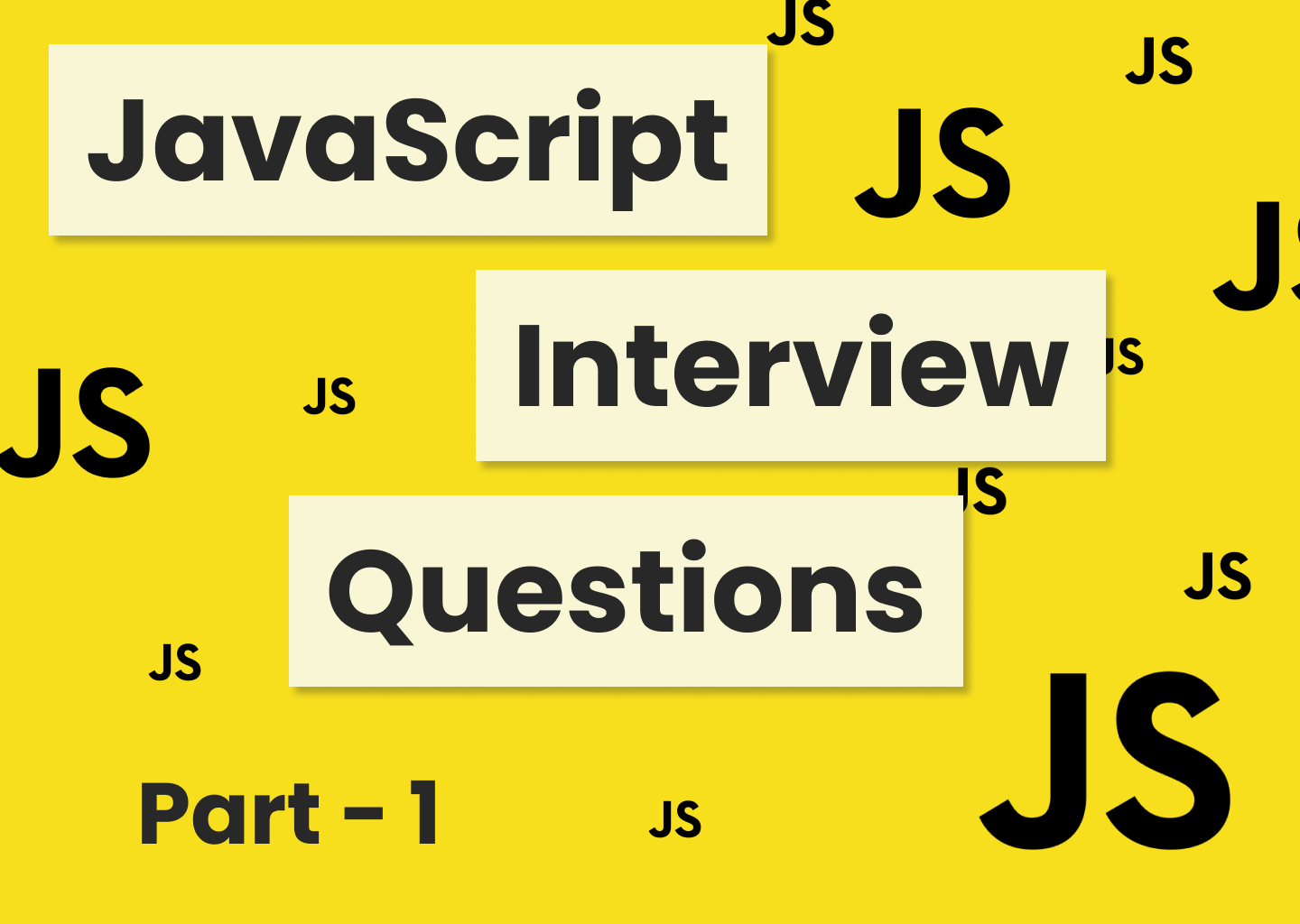
2024-03-25
10 min read
2024-03-25
10 min read
In this post I will list some common JavaScript interview questions. This is the sieries of posts, in which I’will list most commont questions with answers and explanations to them. I offer you to try to answer the question by yourself and then look into the answer
With a given array of users write the code to:
1// Array of users2const users = [3 {4 id: 1,5 name: "Jack",6 isActive: true,7 age: 20,8 },9 {10 id: 2,11 name: "John",12 isActive: true,13 age: 18,14 },15 {16 id: 3,17 name: "Mike",18 isActive: false,19 age: 30,20 },21];
1// Answer2const neededUsers = users3 .sort((user1, user2) => (user1.age < user2.age ? 1 : -1))4 .filter((user) => user.isActive)5 .map((user) => user.name);6
7console.log(neededUsers);
All wee need to do is use basic javascript methods:
.sort() : callback function takes two arguments - in our case user1 and user2 and compares them using ternay operator. If the result of our evaluation is true it returns 1 and if false -1. This way users are sorted in descending order.
.filter(): iterates over the array and returns a new array of users which have isActive = true;
.map(): maps over the array and applies a callback function to each item in array: in our case just returns a name of a user.
I chained all methods but it’s absolutely ok to write each one separately and refactor later.
MDN Map
MDN Filter
MDN Sort
What will be logged to the console in first and second examples?
1// Example 12let var1;3console.log(var1);4console.log(typeof var1);
1// Answer2undefined;3`undefined`;
Declared but anassigned variable in js has ‘undefiend’ value and ‘undefined’ type. (typeof operator returns operand`s type as a tring)
1// Example 22let var2 = null;3console.log(var2);4console.log(typeof var2);
1// Answer2null;3[object]; // "object" (not "null" for legacy reasons)
Null and undefined in JS are both used to descirbe absence of a meaningfull value. Undefined usually used by JS engine, and null is used py programmer to explicitly show that variable doesn`t have any meaningfull value;
MDN Null
MDN Undefined
What will be console logged ?
1//Q12console.log(foo);3foo = 1;
1// Answer2reference Error
“foo = 1” - is a undeclared variable . In strict mode it throws the reference error, in non-strict mode it is a global variable the same as var and will console log ‘undefined’.
1//Q22console.log(foo);3var foo = 2;
1// Answer2Undefined;
var declaration of a variable in JS is hoisted to the top of the scope. But not it`s assigned value.
MDN Hoisting
FreeCodeCamp Post
Task: Create a counter function which has increment and getValue functionality.
1// Answer2function privateCounter() {3 let count = 0;4 return {5 increment: (value = 1) => {6 count += value;7 },8 getValue: () => {9 return count;10 },11 };12}13
14const counter = privateCounter();15
16console.log(counter.getValue());17counter.increment();18console.log(counter.getValue());
A closure is the combination of a function and a lexical environment within which that function was declared. Closures are functions that have access to the outer(enclosing) function’s variables, even after the outer function was returned.
In our solution access to the ‘count’ variable is only possible through counter methods, we cannot access it in any other way. It’s, so to say, ‘closed’ inside our counter function. Used in data privacy and more.
JsInfo Closure Article
MDN Closure
Task: Write a function which helps to achieve multiply(a)(b) and returns product of a and b.
1// Answer2function multiply(a) {3 return (b) => {4 return a * b;5 };6}7const val = multiply(2)(4);8console.log(val);
Currying is a technique in JavaScript that allows you to transform functions with multiple arguments into a sequence of functions, each taking one argument at a time. It promotes code reusability, composability, and flexibility
JsInfo Currying
FreeCodeCamp Post Currying
Task: Write a function which gets an array and an element and returns an array with this element in the end.
1// Answer2const nums = [1, 2];3
4function moveToTheEnd(arr, el) {5 const newArr = [...arr, el];6 return newArr;7} // This is also called a pure function: a) returns same result with the same input; b) doesn`t modify any variables outside the function.8console.log(moveToTheEnd(nums, 9)); // [1, 2, 9]9console.log(nums); // [1,2]10
11// Also you can write it in one line using arrow function:12const addToTheEnd = (arr, el) => [...arr, el];
All we need to do is to use spread operator(…) which ‘spreads’ or destructures and makes a copy of the items in the array.
Task: Write a function which will concatenate 2 arrays.
1const array1 = [1, 2];2const array2 = [3, 4];3
4// Answer 1: using spread operator5function concatArrs(arr1, arr2) {6 return [...arr1, ...arr2];7}8
9// Answer 2: using concat method10function concatArrs2(arr1, arr2) {11 return arr1.concat(arr2);12}13
14console.log(concatArrs(array1, array2));15console.log(concatArrs2(array1, array2));
Again we can solve this with spread operator or with .concat() method.
JsInfo Spread and Rest syntax
MDN Spread syntax
Task: Check that user with given name exists in array of objects.
1// Name to find: Mike2const users = [3 {4 id: 1,5 name: "Jack",6 isActive: true,7 age: 20,8 },9 {10 id: 2,11 name: "John",12 isActive: true,13 age: 18,14 },15 {16 id: 3,17 name: "Mike",18 isActive: false,19 age: 30,20 },21];
1const users = [2 {3 id: 1,4 name: "Jack",5 isActive: true,6 age: 20,7 },8 {9 id: 2,10 name: "John",11 isActive: true,12 age: 18,13 },14 {15 id: 3,16 name: "Mike",17 isActive: false,18 age: 30,19 },20];21
22// Answer 1: usign for-of loop23const findName = (userName, users) => {24 let exists = false;25 for (let user of users) {26 if (user.name === userName) exists = true;27 }28 return exists;29};30
31console.log(findName("Mike", users));32
33// Answer 2: using .some() method34const isNameExists = (userName, arr) =>35 arr.some((user) => user.name.toLowerCase() === userName.toLowerCase());36console.log(isNameExists("Kike", users));37
38// Answer 3: using findIndex39const isNameExists2 = (userName, arr) => {40 // If not found, .findIndex() will return -141 const index = arr.findIndex((user) => user.name === userName);42
43 return index >= 0;44};
Here we have three different ways(and probably more) to solve this. Any of those will do the job, just pick up the one that you like and understand and use it.
for…of loop: declaring the variable ‘exists’ with boolean value type, mapping over the array and comparing each user’s name property with userName we need. If found - sets the exists to true and end the loop.
.some() method: probably fits the best for this task, maps over the array and looks for what we need. Returns true/false;
findIndex() method: maps over users in array, comparing the userName to name property, if found - returns the index of the item, if not - returns -1;
MDN for…of loop
MDN .findindex()
MDN .Some()
Task: Remove all duplicates in the array.
1const array = [1, 2, 2, 3, 4, 5, 5, 5, 6, 7];
1const array = [1, 2, 2, 3, 4, 5, 5, 5, 6, 7];2//Answer 1: using new Set(arr)3const uniqueArr = (arr) => {4 return [...new Set(arr)];5};6console.log(uniqueArr(array));7
8//Answer 2: ForEach loop9const uniqueArr2 = (arr) => {10 const res = [];11 arr.forEach((item) => {12 if (!res.includes(item)) {13 res.push(item);14 }15 });16 return res;17};18
19//Answer 3: .reduce()20const uniqueArr3 = (arr) => {21 return arr.reduce((acc, el) => {22 return acc.includes(el) ? acc : [...acc, el];23 }, []);24};
The solutions:
MDN Set
MDN .forEach
MDN .reduce()
Task 1: Sort the array of numbers.
1const array = [2, 10, 19, -12, 32, 11, 29, -91];
1// Answer2const array = [2, 10, 19, -12, 32, 11, 29, -91];3
4// Mutating the origial array5console.log(array.sort((a, b) => a - b));6
7// Without mutating8const newSortedArray = array.toSorted((a, b) => a - b);9console.log(newSortedArray);
array.sort((a, b) => a - b) sorts the array using a comparison function. In this case, the comparison function subtracts b from a.
array.toSorted() works the same pretty much, but makes a copy of array, without mutating the original. Added in 2023, safe to use in modern browsers already.
Task 2: Sort array of objects by athors last name.
1const books = [2 { name: "Harry Potter", author: "Joanne Rowling" },3 { name: "Warcross", author: "Marie Lu" },4 { name: "The Hunger Games", author: "Suzanne Collins" },5];
1// Answer2const books = [3 { name: "Harry Potter", author: "Joanne Rowling" },4 { name: "Warcross", author: "Marie Lu" },5 { name: "The Hunger Games", author: "Suzanne Collins" },6];7
8books.sort((book1, book2) => {9 const authorLastName1 = book1.author.split(" ")[1];10
11 const authorLastName2 = book2.author.split(" ")[1];12
13 return authorLastName1 < authorLastName2 ? -1 : 1;14});15console.log(books);
Solution: splitting the fullName to get last name of the author; If authorLastName1 is lexicographically less than authorLastName2, it returns -1, otherwise it returns 1, indicating that book2 should come before book1 in the sorted array.
MDN .sort()
MDN .toSorted()
Here are 10 of most common JavaScript interview coding questions you can face in 2024. This is a series of interview preperation posts. So stay tuned! You can follow me on social media, where I’ll introduce new blog posts as soon as they are published on the website.