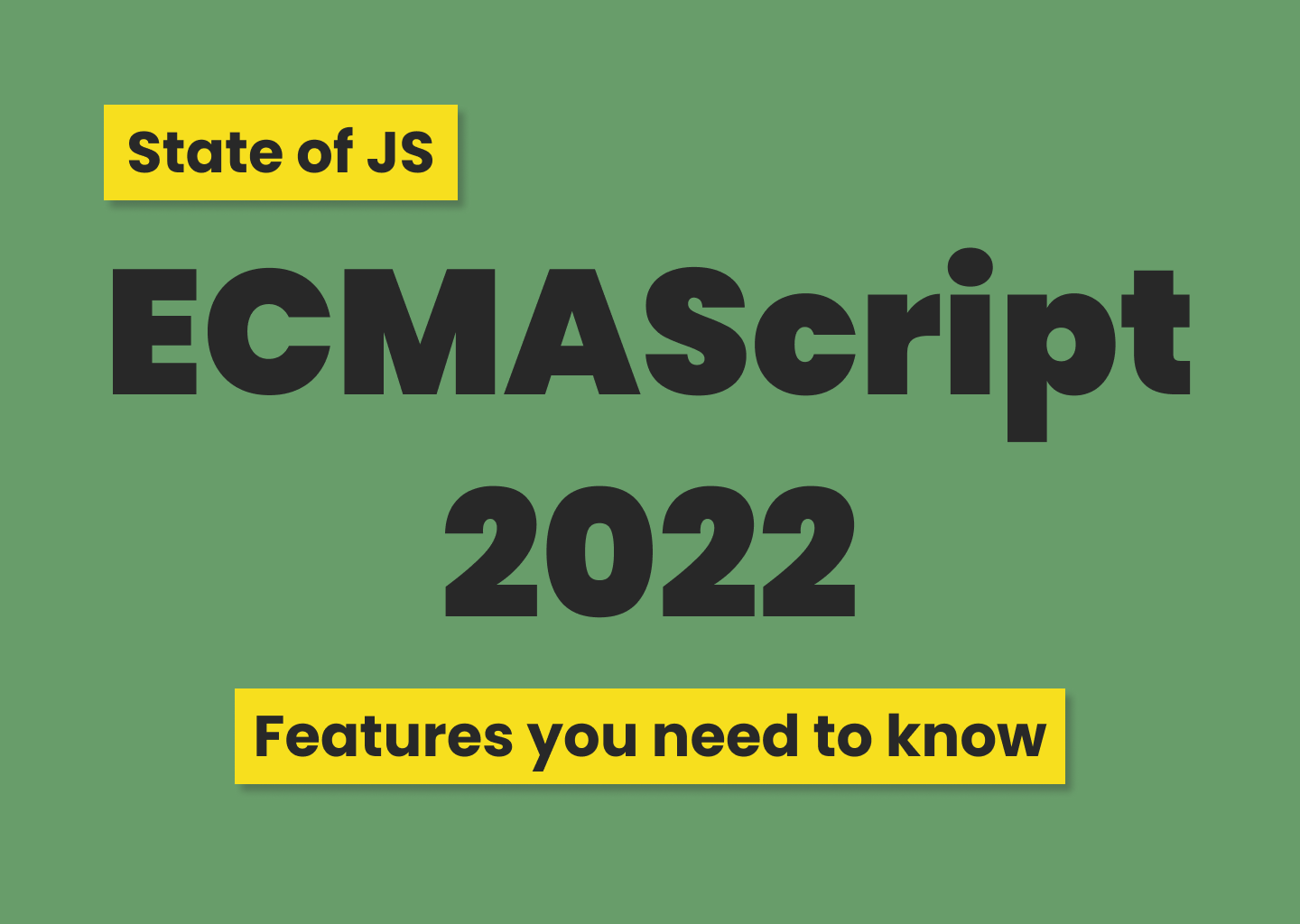
2024-03-27
7 min read
2024-03-27
7 min read
JavaScript, the ubiquitous programming language that powers the web, underwent a remarkable evolution in 2022. The updates introduced during this year have significantly enhanced the language’s capabilities, catering to the ever-growing demands of modern web development. In this comprehensive guide, we’ll delve deep into each of the prominent features added to JavaScript in 2022, providing in-depth explanations and practical examples to help you harness their full potential in your projects.
One of the most anticipated and game-changing features introduced in JavaScript is the implementation of top-level await. Prior to this addition, developers were required to wrap asynchronous operations within an async function to utilize the await keyword. This often led to a proliferation of nested async functions, resulting in complex and harder-to-maintain code structures.
With top-level await, the landscape has changed dramatically. Developers can now leverage the await keyword directly at the module level, without the need for an enclosing async function. This simplifies the handling of asynchronous tasks and allows for a more linear and readable code flow. Consider the following example:
1// Before top-level await2async function fetchData() {3 const response = await fetch("https://api.example.com/data");4 const data = await response.json();5 return data;6}7
8const data = await fetchData();9console.log(data);
_
1// After top-level await2const response = await fetch("https://api.example.com/data");3const data = await response.json();4console.log(data);
In the updated code, the asynchronous fetch operation and the subsequent JSON parsing are now directly wrapped in await statements, without the need for an additional async function. This streamlined approach enhances code readability and makes it easier to reason about the flow of execution.
Encapsulation and data hiding are fundamental principles in object-oriented programming, and JavaScript has now embraced these concepts with the introduction of private class fields and methods. Prior to this addition, developers had to rely on various conventions, such as naming private members with a leading underscore, to denote their intended privacy.
With the new private class fields and methods, developers can now explicitly mark members as private using the # prefix. These private members are accessible only within the class they are defined in, ensuring that the internal implementation details are hidden from the outside world.
1class MyClass {2 #privateField = 10;3
4 #privateMethod() {5 return this.#privateField;6 }7
8 getPrivateField() {9 return this.#privateMethod();10 }11}12
13const instance = new MyClass();14console.log(instance.getPrivateField()); // Output: 1015console.log(instance.#privateField); // Error: Private field '#privateField' is not defined
In this example, the #privateField and #privateMethod are private members of the MyClass. They can only be accessed and used within the class itself, ensuring a higher level of encapsulation and data protection.
Accessing elements in strings, arrays, and typed arrays is a common task in JavaScript development. The introduction of the at() method simplifies this process by providing a more intuitive and versatile way to retrieve elements, especially those at the end of the collection.
Prior to the at() method, developers had to rely on indexing using square brackets, which could become cumbersome when dealing with negative indices to access the last element. The at() method now allows you to use both positive and negative indices to retrieve the desired element, making your code more expressive and easier to read.
1const myArray = [1, 2, 3, 4, 5];2console.log(myArray.at(-1)); // Output: 53
4const myString = "Hello";5console.log(myString.at(-1)); // Output: "o"
In the example above, the at(-1) method is used to access the last element of the array and the last character of the string, respectively. This approach is more intuitive and self-explanatory than the traditional indexing method.
Trailing commas, a feature previously introduced for array and object literals, have now been extended to function parameter lists and function calls. This addition enhances code readability and simplifies the process of maintaining and modifying function signatures, especially when dealing with long parameter lists.
Before the introduction of trailing commas in function parameters, adding or removing a parameter would often result in a need to update the comma placement in the surrounding code. With the new feature, this tedious task is greatly simplified, as the commas can now be left in place, making the code more maintainable and less prone to errors.
1function myFunction(param1, param2) {2 // Function body3}4
5myFunction("a", "b");
In this example, the trailing commas in both the function declaration and the function call make it easier to add, remove, or rearrange parameters without introducing unnecessary changes to the surrounding code.
The Object.hasOwn() method is a welcomed addition to the JavaScript ecosystem, providing a safer and more reliable way to check if an object has a specific property as its own (not inherited) property. This method serves as a replacement for the traditional hasOwnProperty() method, which can be problematic in certain scenarios where the object’s own properties may be overridden.
The Object.hasOwn() method ensures that the property check is performed directly on the object itself, without the risk of encountering issues related to property shadowing or the object’s prototype chain. This enhancement helps developers write more robust and reliable code, especially when working with complex object structures.
1const myObject = {2 prop: "value",3};4
5console.log(Object.hasOwn(myObject, "prop")); // Output: true6console.log(Object.hasOwn(myObject, "toString")); // Output: false
In this example, the Object.hasOwn() method correctly identifies that the myObject has the ‘prop’ property as its own, but it does not have the ‘toString’ property as its own (it is inherited from the object prototype).
JavaScript’s support for classes has been steadily improving, and the introduction of static class blocks is a significant enhancement. This feature allows developers to define static initialization blocks within classes, facilitating the initialization of static class members.
Prior to this addition, the initialization of static class members often required the use of complex class expressions or placement of the initialization logic outside the class definition. Static class blocks provide a more organized and expressive way to handle this task, improving code readability and maintainability.
1class MyClass {2 static #config;3
4 static {5 // Initialize static configuration6 MyClass.#config = {7 key: "value",8 };9 }10}
In this example, the static class block is used to initialize the #config static property of the MyClass. This approach keeps the static member initialization logic encapsulated within the class, making the code more self-contained and easier to understand.
Handling and debugging errors is a crucial aspect of software development, and the introduction of the “Error Cause” feature in JavaScript enhances this process. The Error constructor now accepts an options object with a cause property, allowing developers to create chained error objects that provide more context about the error’s origin.
This feature enables the creation of a hierarchical error structure, where the “main” error can be associated with the “cause” error. This additional information can be invaluable during the debugging process, as it helps developers better understand the root cause of the issue and trace the error’s propagation through the codebase.
1const causeError = new Error("Cause of the error");2const mainError = new Error("Main error", { cause: causeError });3
4console.error(mainError);
In this example, the mainError object is created with the cause option set to the causeError object. When the mainError is logged, it will provide information about both the “main” error and the “cause” error, making it easier to identify and resolve the underlying issue.
The JavaScript features added in 2022 represent a significant leap forward for the language, empowering developers to write more concise, readable, and maintainable code. By exploring and mastering these features, you can enhance your development workflow, improve code and stay up to date with the lates JS features.